Share
Reddit
Twitter
Pinterest
Facebook
Google+
StumbleUpon
Tumblr
Delicious
Digg
Setting up ASP.NET MVC 4 and Twitter Bootstrap 3 with LESS
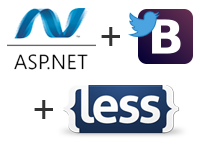
Setup
First, make sure you have the following NuGet packages installed:
Dependencies:- Twitter.Bootstrap.Less
- BundleTransformer.Less
- JavaScriptEngineSwitcher.Msie
- BundleTransformer.MicrosoftAjax
In the RegisterBundles(BundleCollection bundles) function of the BundleConfig class, add this block of code:
App_Start/BundleConfig.cs#region LESS
var nullOrderer = new NullOrderer();
var css = new CustomStyleBundle("~/Content/less").Include("~/Content/bootstrap/bootstrap.less");
css.Orderer = nullOrderer;
bundles.Add(css);
#endregion
Be sure to include the new bundle in the <head> tag of your layout view:
Views/Shared/_Layout.cshtml@Styles.Render("~/Content/less")
Also, some changes will need to be made to the configuration/bundleTransformer section in the Web.config. The defaultMinifier will need to be set for css and js minification and the less/jsEngine property will also need to be added.
Web.config<configuration>
<bundleTransformer xmlns="http://tempuri.org/BundleTransformer.Configuration.xsd">
...
<css defaultMinifier="MicrosoftAjaxCssMinifier">
...
<js defaultMinifier="MicrosoftAjaxJsMinifier">
...
<less>
<jsEngine name="MsieJsEngine" />
</less>
...
</bundleTransformer>
</configuration>
When you are testing this setup, it may be helpful to add the following line to the end of the RegisterBundles function in your BundleConfig if you'd like to verify the minification process in a development environment:
App_Start/BundleConfig.csBundleTable.EnableOptimizations = true;
Remember that you can also control which environments are deployed with the minified versions versus the original LESS files by using Web.config Transformation Syntax:
Web.config<system.web>
<compilation debug="true" />
</system.web>
Web.Release.config
<system.web>
<compilation xdt:Transform="RemoveAttributes(debug)" />
</system.web>
That's all! Thanks for reading. Good luck and enjoy using Twitter Bootstrap (with LESS) in ASP.Net MVC.
References:
Update: As Andrey pointed out in the comments, it is unnecessary and not recommended to use both BundleTransformer and CssMinify. After verifying that the code still minified the CSS correctly, I have updated the post to include the update the code.
Update: Andrey pointed out that since ScriptBundle and StyleBundle already use transformations, it is recommended to instead use the Bundle Transformer class "CustomStyleBundle". Also, when switching to the recommended CustomStyleBundle, I noticed the LESS code wasn't minifying anymore (although it was compiling to CSS), so I added the BundleTransformer.MicrosoftAjax NuGet package to minify the translated LESS and specified in the <bundleTransformer> section of the Web.Config the MicrosoftAjax adapters as the defaultMinifiers. I edited the post to update the code sections and the explanations.